Hi Folks,
I've been trying since a long time finding an article of how to pass parameters to a Report Viewer control in Visual Studio 2010, but most of the articles needs lots of code to be written.
But, now I have found the way to create a report and pass parameters easily with only 1 line of code, yes I am serious 1 line of code!! :)
And below I will describe the steps with screen shots:
1- You need to create a new Visual Studio Web Site:
2- Then you will have an empty web site.
3- Now, I will create a new database with one table with three columns (ID, Name, City):
Then fill it with some data:
Now I will create a data set for the Contacts table:
Now I will Rebuild the web site:
Now, we need to add reference to Microsoft.ReportViewer.WebForms:
Make sure to select Version 10.0.0.0
Now, I will create a report:
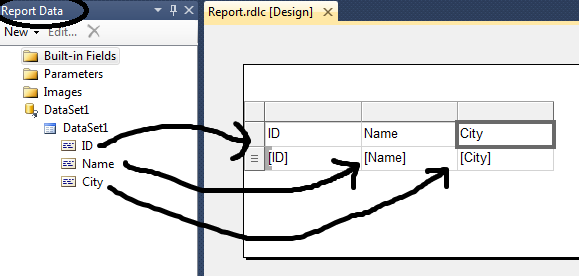
Save the report.
Now, I will start to build the page of the report:
- Add a DropdownList and a Button:
- Drop a Script Manager control into the page:
- Drop a ReportViewer control:
- Choose the Report we created before, and an ObjectDataSource will be created directly:
- On the Click event of the button write the only Code you need to write to refresh the report:
ReportViewer1.LocalReport.Refresh()
Congratulations!! the web site is ready and the report takes the parameter and refreshes.
Happy programming!
Saed
I've been trying since a long time finding an article of how to pass parameters to a Report Viewer control in Visual Studio 2010, but most of the articles needs lots of code to be written.
But, now I have found the way to create a report and pass parameters easily with only 1 line of code, yes I am serious 1 line of code!! :)
And below I will describe the steps with screen shots:
1- You need to create a new Visual Studio Web Site:
2- Then you will have an empty web site.
3- Now, I will create a new database with one table with three columns (ID, Name, City):
Then fill it with some data:
Now I will create a data set for the Contacts table:
Now I will Rebuild the web site:
Now, we need to add reference to Microsoft.ReportViewer.WebForms:
Make sure to select Version 10.0.0.0
Now, I will create a report:
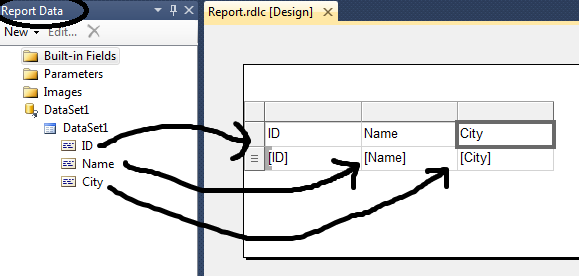
Save the report.
Now, I will start to build the page of the report:
- Add a DropdownList and a Button:
- Drop a Script Manager control into the page:
- Drop a ReportViewer control:
- Choose the Report we created before, and an ObjectDataSource will be created directly:
- On the Click event of the button write the only Code you need to write to refresh the report:
ReportViewer1.LocalReport.Refresh()
Congratulations!! the web site is ready and the report takes the parameter and refreshes.
Happy programming!
Saed